Nov 06, 2017 This video shows an implementation of, sigh, Flappy Bird in C at the command prompt. Source: https://github.com/OneLoneCoder/videos/blob/master/OneLoneCode. I'm programming a game in C that's supposed to be something like the smartphone game 'Flappy Bird'. You who have played it knows its about avoiding approaching obstacles with gaps in them, with a bird thats constantly moving downwards, and you have to click a button to keep it in the air. How to create a flappy bird tutorial was one of the first tutorial we did on this website, we are back now with a new one where we will show you how to make a new and improved version of flappy bird using Microsoft Visual Studio, WPF and C#. In this game you will have your standard pipes and a gap between them where the bird will fly through.
- #include <string>
- #include <fstream>
- #include <ctime>
- public:
- bird_x =100;
- bird[0].setFillColor(sf::Color::Red);
- bird[1].setFillColor(sf::Color::Yellow);
- bird[1].setPosition(bird_x +71, bird_y +20);
- bird.push_back(sf::CircleShape(3.f));
- bird[2].setPosition(bird_x +20, bird_y +10);
- void getPosition(float*x, float*y){
- *y = bird_y +15;
- void moveVertically(float mov){
- for(unsignedint i =0; i < bird.size(); i++){
- }
- std::vector<sf::CircleShape> getBirdVector(){
- }
- std::vector<sf::CircleShape> bird;
- float bird_y;
- public:
- Pipe(unsignedint space, unsignedint window_width, unsignedint window_height){
- printf('Spawning new pipe with gap from %u to %un', space -100, space +100);
- upper_pipe =new sf::RectangleShape(sf::Vector2f(100, space -100));
- lower_pipe =new sf::RectangleShape(sf::Vector2f(100, window_height - space -100));
- upper_pipe->setFillColor(sf::Color::Green);
- controlled_space = space;
- upper_pipe->setPosition(current_x, 0);
- }
- bool checkIfCollision(float colliding_x, float colliding_y){
- if(colliding_x > current_x +50|| colliding_x < current_x -50)returnfalse;
- if(colliding_y < controlled_space +100&& colliding_y > controlled_space -100)returnfalse;
- }
- if(current_x <-100)returntrue;
- upper_pipe->move(-1, 0);
- returnfalse;
- void drawSelf(sf::RenderWindow*to_draw_to){
- to_draw_to->draw(*lower_pipe);
- }
- delete upper_pipe;
- }
- private:
- sf::RectangleShape*lower_pipe;
- unsignedint controlled_space;
- char*name =(char*)calloc(1, 1);
- printf('Enter your name: ');
- scanf('%c', &buf);
- name =(char*)realloc(name, strlen(name)+2);
- }
- savefile.open('.flappy_bird_save', std::ios::out| std::ios::app);
- savefile <<':';
- savefile.write((char*)(void*)(&score), sizeof(unsignedint));
- savefile.close();
- printf('LEADERBOARDn');
- unsignedint score;
- };
- char buf =0;
- while(savefile.good()){
- players.resize(players.size()+1);
- players[players.size()-1].name=(char*)calloc(1, 1);
- buf = savefile.get();
- players[players.size()-1].name=(char*)realloc(players[players.size()-1].name, strlen(players[players.size()-1].name)+2);
- players[players.size()-1].name[strlen(players[players.size()-1].name)]= buf;
- savefile.read((char*)(void*)&(players[players.size()-1].score), sizeof(unsignedint));
- }
- if(players.size()<3)return;//don't bother
- for(unsignedint i =0; i < players.size(); i++){
- if(players[pivot_pos].score0) pivot_pos -=1;
- if(players[i].score< players[pivot_pos].score){
- players.erase(players.begin()+ i);
- }
- for(unsignedint i =0; i < players.size()-2; i++){
- is_sorted =false;
- }
- if(is_sorted)break;
- if(players[i].score0)break;
- printf('%s:t%8un', players[i].name, players[i].score);
- }
- if(argc <2)return1;
- std::default_random_engine rand_generator;
- printf('Insert quarter to playn');
- unsignedint window_width =(argc <3)?1000:atoi(argv[2]);//we have to do it this way because SFML team is too lazy to let you
- unsignedint window_height =(argc <4)?800:atoi(argv[3]);//actually get the size of the window.
- sf::RenderWindow window(sf::VideoMode(window_width, window_height), argv[1]);//damn kids...
- std::uniform_int_distribution<int> pipe_pos_spawn(100, window_height -100);
- Bird protagonist;
- std::vector<Pipe> pipes;
- float protagonist_x;
- char*score_text =(char*)calloc(1, 11);
- sf::Time check_time;
- sf::RectangleShape*current_upper_pipe;
- if(!font.loadFromFile('Hack-Regular.ttf'))return1;
- score_view.setCharacterSize(32);
- score_view.setFillColor(sf::Color::White);
- score_view.setString('Score: '+ std::string(score_text));
- printf('Scoreview is currently %sn', score_view.getString().toAnsiString().c_str());
- pipe_pos_spawn(rand_generator);//throwaway first value
- protagonist.moveVertically(window_height /2);
- while(window.isOpen()){
- while(window.pollEvent(event)){
- if(event.type sf::Event::Closed) window.close();
- protagonist.getPosition(&protagonist_x, &protagonist_y);
- current_gravity_pull =(current_gravity_pull *2<16)? current_gravity_pull :16;
- if(sf::Keyboard::isKeyPressed(sf::Keyboard::Space)&& protagonist_y >0){
- protagonist.moveVertically(-2);
- protagonist.moveVertically(current_gravity_pull);
- if(check_time.asMilliseconds()%10000){
- pipes.push_back(Pipe(pipe_pos_spawn(rand_generator), window_width, window_height));
- for(unsignedint i =0; i < protagonist_parts.size(); i++){//draw the bird
- }
- pipes[i].drawSelf(&window);
- printf('Update returned truen');
- pipes[i].~Pipe();
- score +=1;
- score_view.setString(std::string(score_text));
- printf('Scoreview is currently %sn', score_view.getString().toAnsiString().c_str());
- }elseif(pipes[i].checkIfCollision(protagonist_x, protagonist_y)|| protagonist_y >= window_height){
- window.close();
- return0;
- }
- window.display();
- return0;
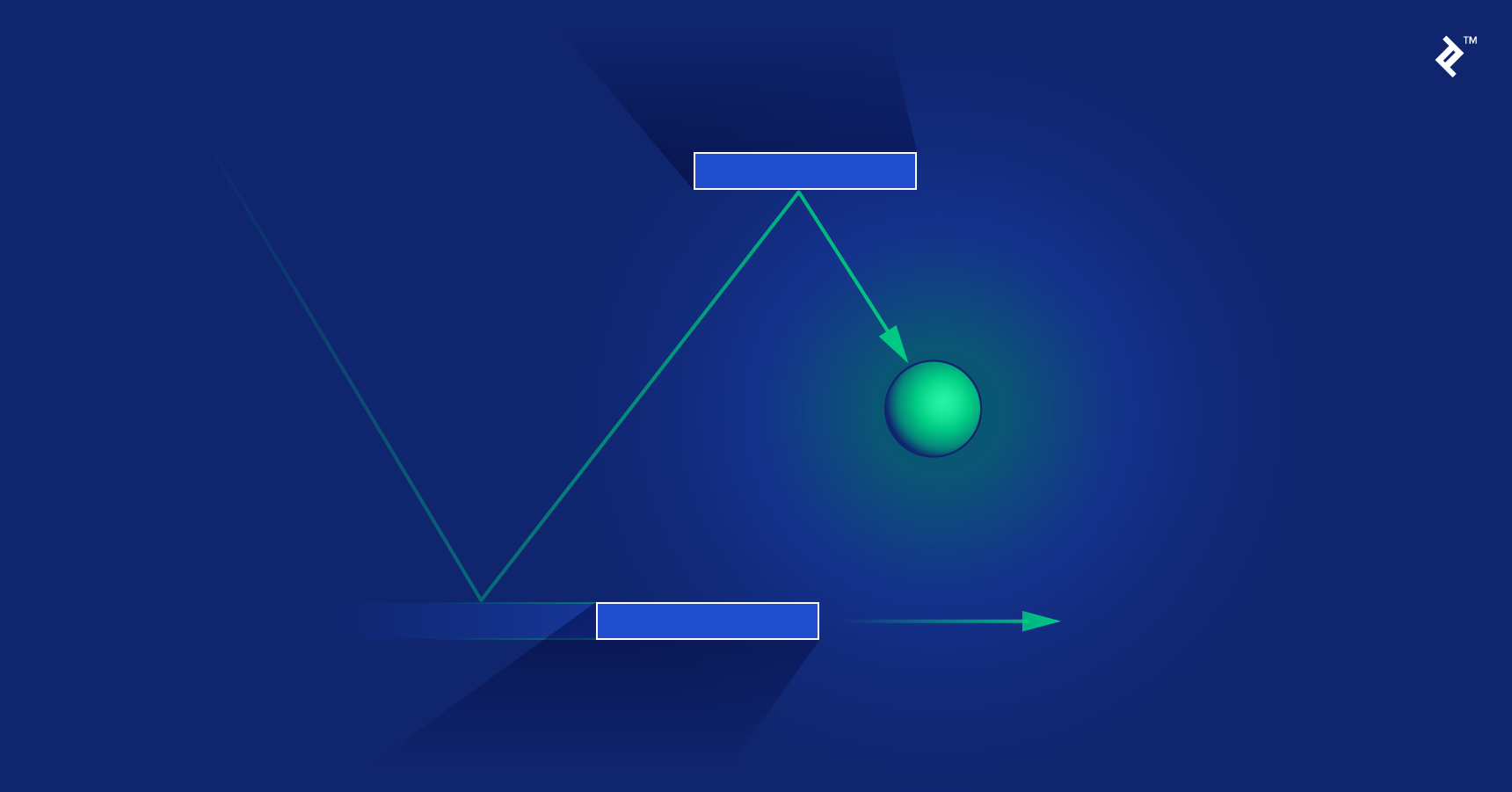
- Sep 27, 2015 Flappy Bird in C (VS 2013). GitHub Gist: instantly share code, notes, and snippets. Skip to content. All gists Back to GitHub. Sign in Sign up Instantly share code, notes, and snippets. Soachishti / CPP-Flappy-Bird.cpp.
- The most accurate Flappy Bird clone out there. Made with love. Free to play, NOW! Mister Moonboots. Free game for the iPad by Mr Speaker: Like bouncing? The infinity of space?
- I wrote the code myself with Code.org.
Join GitHub today
GitHub is home to over 40 million developers working together to host and review code, manage projects, and build software together.
Sign up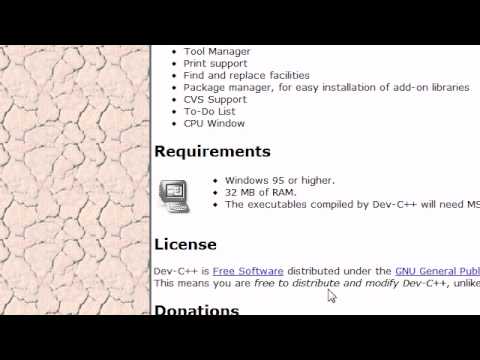
Branch:master
Program Flappy Bird
#include<SFML/Graphics.hpp> |
#include<SFMLAudio.hpp> |
#include<iostream> |
#include<cstdlib> |
#include<ctime> |
#include'Pipes.h' |
#include'GameOver.h' |
Pipes::Pipes(int PositionX, int PositionY, int PipeDistance, int PipeAbove) |
{ |
std::cout << 'hello!' << std::endl; |
if (!PipeUp.loadFromFile('images/FlappyBird.png', sf::IntRect(84, 323, 26, 160))) |
{ |
} |
if (!PipeDown.loadFromFile('images/FlappyBird.png', sf::IntRect(56, 323, 26, 160))) |
{ |
} |
PipeSpriteUp.setTexture(PipeUp); |
PipeSpriteUp.setScale(4,4); |
PipeSpriteDown.setTexture(PipeDown); |
PipeSpriteDown.setScale(4, 4); |
PipeSpriteUp.setPosition(PositionX + PipeDistance, PositionY*1.4 ); |
PipeSpriteDown.setPosition(PositionX + PipeDistance, PositionY - PipeAbove + 160); |
PipeGap = PipeAbove; |
} |
Pipes::~Pipes() |
{ |
} |
voidPipes::draw(sf::RenderWindow &window) |
{ |
//srand((unsigned)time(NULL)); |
PipeSpriteUp.move(Speed, 0); |
PipeSpriteDown.move(Speed, 0); |
if (PipeSpriteUp.getPosition().x <= -100 && PipeSpriteDown.getPosition().x <= -100) |
{ |
int Up = rand() % (600 + 1 - 300) + 300; |
int Down = Up - PipeGap; |
PipeSpriteUp.setPosition(800, Up); |
PipeSpriteDown.setPosition(800, Down); |
} |
window.draw(PipeSpriteUp); |
window.draw(PipeSpriteDown); |
} |
intPipes::OverlapTest(float FlappyBirdX, float FlappyBirdY) |
{ |
constint left_box0 = FlappyBirdX; |
constint right_box0 = FlappyBirdX + 17 * 3; |
constint top_box0 = FlappyBirdY; |
constint bottom_box0 = FlappyBirdY + 12 * 3; |
constint left_box1 = PipeSpriteUp.getPosition().x; |
constint right_box1 = PipeSpriteUp.getPosition().x + 26 * 4; |
constint top_box1 = PipeSpriteUp.getPosition().y; |
constint bottom_box1 = PipeSpriteUp.getPosition().y + 160 * 4; |
constint left_box2 = PipeSpriteDown.getPosition().x; |
constint right_box2 = PipeSpriteDown.getPosition().x + 26 * 4; |
constint top_box2 = PipeSpriteDown.getPosition().y; |
constint bottom_box2 = PipeSpriteDown.getPosition().y + 160 * 4; |
return ((right_box0 >= left_box1 && left_box0 <= right_box1 && top_box0 <= bottom_box1 && bottom_box0 >= top_box1) || (right_box0 >= left_box2 && left_box0 <= right_box2 && top_box0 <= bottom_box2 && bottom_box0 >= top_box2)); |
} |
intPipes::Points(float FlappyBirdX) |
{ |
int left_box0 = FlappyBirdX; |
int right_box0 = FlappyBirdX + 17 * 2; |
int left_box1 = PipeSpriteDown.getPosition().x + 25; |
int right_box1 = PipeSpriteDown.getPosition().x + 26; |
int left_box2 = PipeSpriteUp.getPosition().x + 29; |
int right_box2 = PipeSpriteUp.getPosition().x + 30; |
return ((right_box0 >= left_box1 && left_box0 <= right_box1) || (right_box0 >= left_box2 && left_box0 <= right_box2)); |
} |
Dev C++ Flappy Bird Download
Copy lines Copy permalink